Flutter, developed by Google, is a popular toolkit for creating apps that work on multiple platforms like Android, iOS, web, and desktop. It allows developers to design beautiful and consistent user interfaces (UIs) efficiently. However, just building an app isn’t enough. If your app doesn’t perform smoothly, users might lose interest, uninstall it, or leave bad reviews.
For developers and business owners alike, ensuring your Flutter app is fast, responsive, and efficient is essential. A well-optimized app not only satisfies users but also leads to better engagement, retention, and business growth.
In this blog, we will go through five practical strategies to help you optimize your Flutter app. Whether you are developing your app for the first time or want to improve an existing one, these steps will ensure your app performs seamlessly.
5 Key Ways to Optimize Flutter App Performance
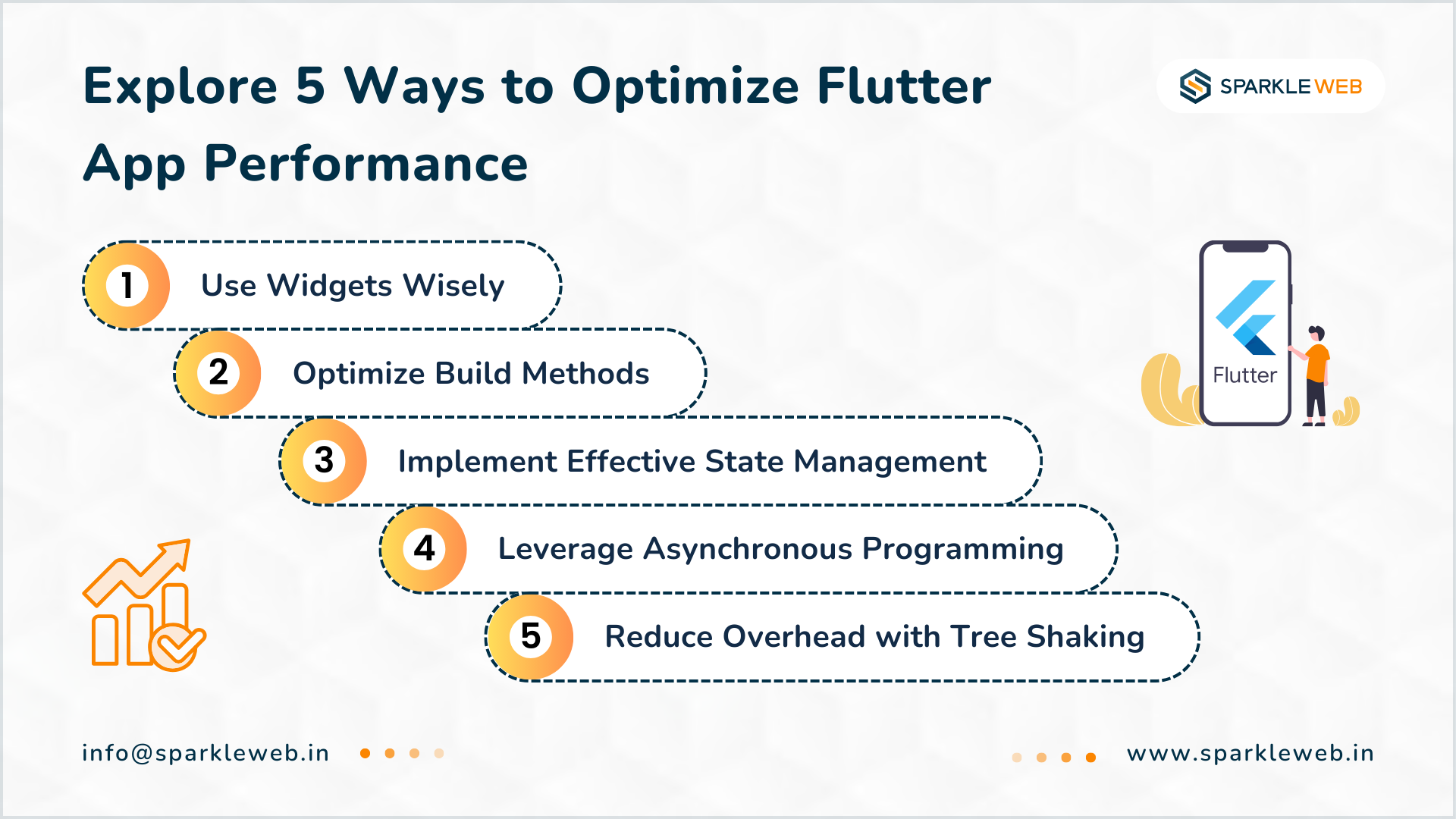
1. Use Widgets Wisely
Widgets are the building blocks of any Flutter app. Everything you see on the screen—text, buttons, images, or containers—is a widget. While widgets offer flexibility and allow developers to create complex designs, improper use of widgets can slow your app down.
Best Practices for Widgets
a. Use Stateless Widgets for Static Content:
If a part of your app’s UI doesn’t change, use a StatelessWidget. This avoids unnecessary rebuilding of the widget, saving processing power.
For example, labels, headings, or icons that remain the same should be in a stateless widget.
Example:
const Text('Welcome to Flutter', style: TextStyle(fontSize: 18));
Using const ensures the widget doesn’t rebuild unnecessarily.
b. Reuse Widgets:
Instead of creating new widgets for the same type of content, reuse existing ones. For instance, if you have multiple similar buttons in your app, create a reusable button widget.
c. Leverage const Constructors:
Adding the const keyword to widgets makes them more efficient because Flutter knows they won’t change and doesn’t have to recreate them during updates.
Impact
By carefully managing widgets, you reduce the time the app spends on rebuilding the UI. This ensures the app runs smoothly without delays or lags.
2. Optimize Build Methods
The build() method in Flutter is called repeatedly, especially during user interactions like scrolling, navigation, or refreshing the UI. If this method takes too long or does too much work, it can make the app feel slow and unresponsive.
Best Practices for Build Methods
a. Avoid Heavy Logic Inside build():
The build() method should only focus on creating the UI. Any data processing, calculations, or API calls should happen outside this method.
b. Break Down Large Widgets into Smaller Components:
If your widget tree becomes too complex, break it into smaller, reusable widgets. This makes the app easier to manage and reduces rebuild times.
c. Use Memoization:
Memoization is a technique where you store the result of a computation so you can reuse it later without recalculating. Flutter has packages like flutter_hooks that make memorization easier.
Example:
Widget build(BuildContext context) {
final data = useMemoized(() => fetchData());
return Text(data);
}
Impact
Optimizing the build() method reduces the load on the app, making navigation and screen loading faster. This improves the overall user experience.
3. Implement Effective State Management
State management is the way you handle the dynamic parts of your app, like user interactions, data updates, and animations. Without proper state management, your app might use too much memory, feel slow, or behave unpredictably.
Recommended State Management Approaches
a. Provider:
This is a simple and lightweight solution suitable for small to medium-sized apps. It helps manage app states efficiently without overcomplicating the code.
b. Riverpod:
Riverpod builds on Provider but offers better performance and flexibility, making it ideal for growing or more complex apps.
c. BLoC (Business Logic Component):
BLoC is useful for apps with advanced requirements. It separates business logic from the UI, ensuring the app stays modular and easy to maintain.
Impact
Good state management ensures your app runs smoothly, avoids glitches during navigation, and uses memory efficiently.
4. Leverage Asynchronous Programming
In any app, certain tasks take time, like fetching data from an API, loading a large file, or running database queries. These tasks can block the app’s main thread, causing the app to freeze temporarily.
How Asynchronous Programming Helps
Flutter allows you to run tasks in the background while keeping the main thread free to handle user interactions.
Best Practices for Asynchronous Programming
a. Use FutureBuilder or StreamBuilder:
These widgets are designed to handle asynchronous data and update the UI once the data is available.
Example:
FutureBuilder(
future: fetchData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator();
} else if (snapshot.hasData) {
return Text(snapshot.data);
} else {
return Text('Error loading data');
}
},
);
b. Optimize API Calls and Queries:
Instead of making multiple calls to an API, batch them or use caching mechanisms to reduce the load.
c. Avoid Blocking the UI with Long Tasks:
Avoid using unnecessary await keywords or running heavy processes on the main thread.
Impact
By using asynchronous programming, your app feels faster and more responsive, even when performing heavy tasks like loading data or saving files.
5. Reduce Overhead with Tree Shaking
Tree shaking is a process that removes unused code from your app. It makes the app smaller and faster to load, especially important for large apps or apps with many dependencies.
How to Enable Tree Shaking
a. Use Optimized Builds:
When creating an APK, use the following command to ensure tree shaking is applied:
flutter build apk --split-per-abi
b. Clean Up Unused Code and Dependencies:
Remove any packages or imports that are no longer used in your app. You can use tools like Dart Pub to check for unused dependencies.
Impact
Tree shaking reduces your app’s size, leading to faster downloads, quicker startup times, and better performance on low-end devices.
Conclusion
Optimizing the performance of your Flutter app is a continuous process that pays off with a better user experience. By using widgets efficiently, improving your build methods, managing state effectively, leveraging asynchronous programming, and applying tree shaking, you can ensure your app performs at its best.
At Sparkle Web, we specialize in Flutter development and performance optimization. Whether you are looking to build a new app or improve an existing one, our team can help you deliver a fast, smooth, and user-friendly experience.
Let’s Work Together! Contact us today to discuss your Flutter app development needs.
Mohit Kokane
A highly skilled Flutter Developer. Committed to delivering efficient, high-quality solutions by simplifying complex projects with technical expertise and innovative thinking.
Reply